برای افزودن placeholder به TextBox در WPF، میتوانید از Adorner یا TextBox با رفتار سفارشی استفاده کنید. در اینجا چند راه برای رسیدن به این هدف وجود دارد:
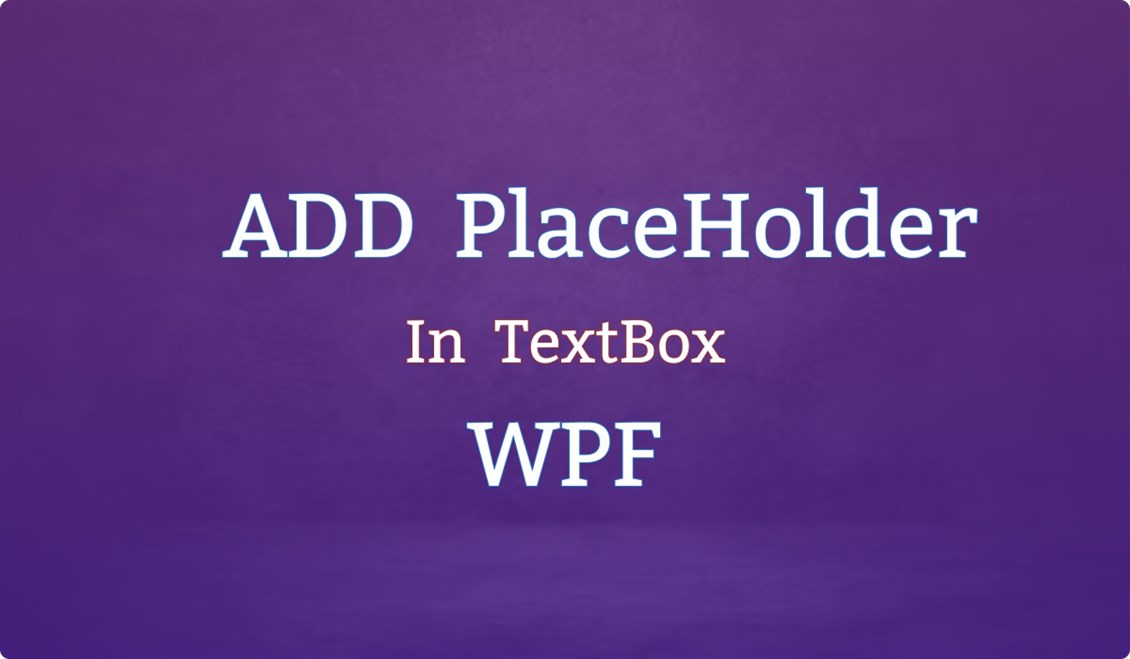
روش 1: استفاده از Adorner
1. یک کلاس PlaceholderAdorner ایجاد کنید:
کلاسی را تعریف کنید که متن محل نگهدارنده را مدیریت کند:
using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; public class PlaceholderAdorner : Adorner { private readonly TextBlock _placeholderTextBlock; public PlaceholderAdorner(UIElement adornedElement, string placeholderText) : base(adornedElement) { _placeholderTextBlock = new TextBlock { Text = placeholderText, Foreground = Brushes.Gray, Margin = new Thickness(5, 0, 0, 0) }; (adornedElement as TextBox).TextChanged += (s, e) => InvalidateVisual(); } protected override void OnRender(DrawingContext drawingContext) { if ((AdornedElement as TextBox).Text.Length == 0) { var desiredSize = _placeholderTextBlock.DesiredSize; drawingContext.DrawText( new FormattedText( _placeholderTextBlock.Text, System.Globalization.CultureInfo.CurrentUICulture, FlowDirection.LeftToRight, new Typeface(_placeholderTextBlock.FontFamily, _placeholderTextBlock.FontStyle, _placeholderTextBlock.FontWeight, _placeholderTextBlock.FontStretch), _placeholderTextBlock.FontSize, _placeholderTextBlock.Foreground ), new Point(5, (AdornedElement.RenderSize.Height - desiredSize.Height) / 2) ); } } }
2. Adorner را به TextBox وصل کنید:
Adorner را به TextBox خود در کد اضافه کنید:
using System.Windows; namespace YourNamespace { public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); var placeholderAdorner = new PlaceholderAdorner(MyTextBox, "Enter text here"); AdornerLayer.GetAdornerLayer(MyTextBox).Add(placeholderAdorner); } } }
3. MainWindow.xaml:
مطمئن شوید که یک TextBox به نام MyTextBox در XAML خود دارید:
<Window x:Class="YourNamespace.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="MainWindow" Height="350" Width="525"> <Grid> <TextBox Width="200" Height="30" Margin="10" Name="MyTextBox"/> </Grid> </Window>
روش 2: کنترل TextBox سفارشی
1. یک کنترل TextBox سفارشی ایجاد کنید:
یک کنترل سفارشی که متن placeholder را مدیریت میکند تعریف کنید:
using System.Windows; using System.Windows.Controls; using System.Windows.Media; public class WatermarkTextBox : TextBox { public static readonly DependencyProperty WatermarkProperty = DependencyProperty.Register("Watermark", typeof(string), typeof(WatermarkTextBox), new PropertyMetadata(string.Empty)); public string Watermark { get { return (string)GetValue(WatermarkProperty); } set { SetValue(WatermarkProperty, value); } } public WatermarkTextBox() { this.GotFocus += RemovePlaceholder; this.LostFocus += ShowPlaceholder; } private void RemovePlaceholder(object sender, RoutedEventArgs e) { if (this.Text == Watermark) { this.Text = string.Empty; this.Foreground = Brushes.Black; } } private void ShowPlaceholder(object sender, RoutedEventArgs e) { if (string.IsNullOrEmpty(this.Text)) { this.Text = Watermark; this.Foreground = Brushes.Gray; } } protected override void OnInitialized(EventArgs e) { base.OnInitialized(e); ShowPlaceholder(null, null); } }
2. از کنترل سفارشی در XAML استفاده کنید:
کنترل سفارشی را به MainWindow.xaml خود اضافه کنید:
<Window x:Class="YourNamespace.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local="clr-namespace:YourNamespace" Title="MainWindow" Height="350" Width="525"> <Grid> <local:WatermarkTextBox Width="200" Height="30" Margin="10" Watermark="Enter text here"/> </Grid> </Window>
با استفاده از روش Adorner یا کنترل TextBox سفارشی، می توانید متن مکان نگهدار را به TextBox در WPF اضافه کنید.